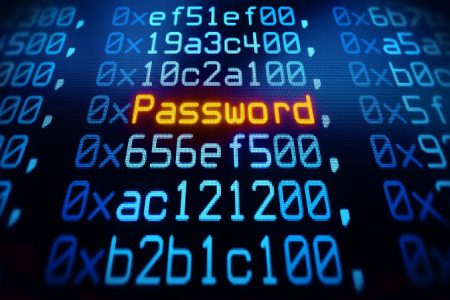
Step-by-Step Guide to Building a Random Password Generator
Password security is a crucial aspect of online safety, yet it often represents one of the weakest links in an individual’s or organization’s cybersecurity defenses. A strong password is one that cannot be easily guessed or cracked through brute force or dictionary attacks. Reusing the same password across multiple accounts is highly risky because it is likely that your long-time password has already been exposed in a data breach, leaving all your accounts vulnerable.To check if your password or email has been compromised, you can use tools like Have I Been Pwned. If you find it cumbersome to change your passwords frequently, you should at least create a long password, with a minimum of 15 characters. Passphrases are particularly effective for this purpose. For example, a passphrase like “Billybobdeclaredhisindependencefromseedoils” is difficult to guess or crack through brute force methods.Now, let’s move on to the code.
#!/usr/bin/python3
import random
import string
def generate_password(length):
# Get a random selection of letters, digits, and punctuation
characters = string.ascii_letters + string.digits + string.punctuation
# Shuffle the characters to get a random selection
characters = random.sample(characters, k=length)
# Join the characters into a single string and return it
return "".join(characters)
# Generate a random password with a length of 13 characters
password = generate_password(13)
print(password)
I created a custom function to encapsulate various actions. This function generates a random selection of letters, digits, and punctuation, shuffles their order, and then joins them together.You can adjust the length of the password by specifying the desired length as an argument in the generate_password(?)
function, where “?” represents the length you want.Below, I’ll break down the code line-by-line:
!/usr/bin/python3
This is the shebang line. On a Unix system, it specifies the Python interpreter for this file.
Import string
Import random
Both of these lines import built-in Python modules that provide essential functionality for our password generator.The random
module is used to generate pseudo-random numbers and perform various random operations. It can also manipulate strings by selecting random elements or shuffling sequences, which is crucial for creating unpredictable passwords.The string
module allows us to easily create strings that include a wide variety of characters. It provides constants for different character sets, such as lowercase and uppercase letters, digits, and punctuation marks. This makes it simple to construct strings that meet specific criteria for password complexity.By importing these modules, we gain access to powerful tools that help us generate secure and random passwords efficiently.
def generate_password(length):
This line defines a function named generate_password
that accepts a single argument, which specifies the desired length of the password to be generated.
# Combine all letters (uppercase and lowercase), digits, and punctuation into a single string
characters = string.ascii_letters + string.digits + string.punctuation
# Select a random sample of characters from the combined string, with the specified length
random_characters = random.sample(characters, k=length)
# Join the randomly selected characters into a single string and return it
return "".join(random_characters)
Line #1 creates a string that includes all letters (both uppercase and lowercase), digits, and punctuation by combining the ascii_letters
, digits
, and punctuation
constants from the string
module.Line #2 uses the random.sample()
function to select a random sample of characters from this string, with the length specified by the generate_password(length)
function.Line #3 joins the selected characters into a single string.
password = generate_password(24)
Now using our function we will generate a 24 character password and store it onto the variable password.
To look up the password we just generated we use the following
print(password)
In conclusion, creating a strong and secure password is a fundamental aspect of maintaining online security. By using a random password generator, you can ensure that your passwords are difficult to guess or crack, thereby enhancing your overall cybersecurity. The custom function we developed demonstrates how to generate a random password by selecting a mix of letters, digits, and punctuation, shuffling them, and joining them into a single string. This method provides a straightforward yet effective way to create robust passwords.Remember, the length and complexity of your password are crucial factors in its strength. Adjusting the length parameter in the generate_password
function allows you to customize the security level of your passwords. Additionally, regularly updating your passwords and using unique passwords for different accounts can further protect you from potential breaches.By following the steps outlined in this guide, you can easily implement your own random password generator and take a proactive approach to safeguarding your digital life. Stay secure and keep your passwords strong!